When managing content-heavy WordPress sites, effective navigation is crucial for a seamless user experience. Pagination plays a vital role in ensuring visitors can browse your content effortlessly. This guide walks you through different types of pagination implementations in WordPress, complete with code snippets and explanations.
Table of Contents
ToggleUnderstanding the Basics of Pagination in WordPress
Before diving into code, here’s a quick overview of the key concepts:
- The Loop: WordPress’s main function for displaying posts. It checks if posts exist and displays each one.
- Pagination: Typically placed after the
endwhile;
statement in the loop to ensure navigation links are displayed after all posts. - No Posts Found: A fallback message displayed when no posts are available.
1. Basic Next and Previous Links
<div class="navigation">
<?php previous_post_link('<div class="prev-link">%link</div>', 'Previous Post: %title'); ?>
<?php next_post_link('<div class="next-link">%link</div>', 'Next Post: %title'); ?>
</div>
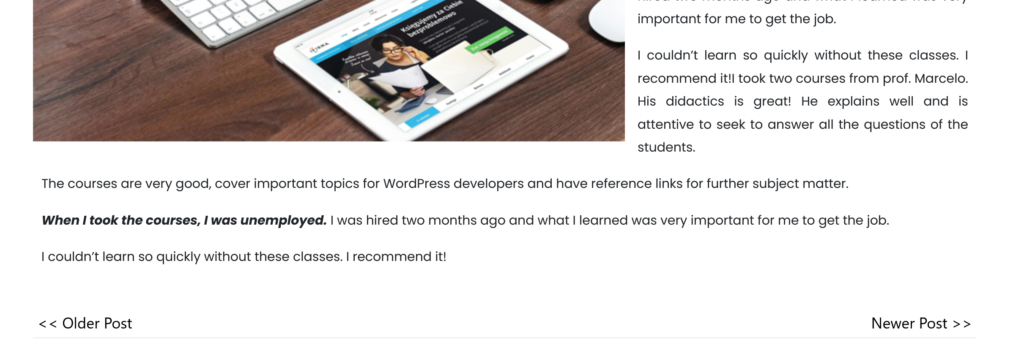
2. Displaying Post Titles in Navigation
<div class="post-navigation">
<?php
if (get_previous_post()) {
previous_post_link('<div class="prev-post">%link</div>', '%title');
}
if (get_next_post()) {
next_post_link('<div class="next-post">%link</div>', '%title');
}
?>
</div>
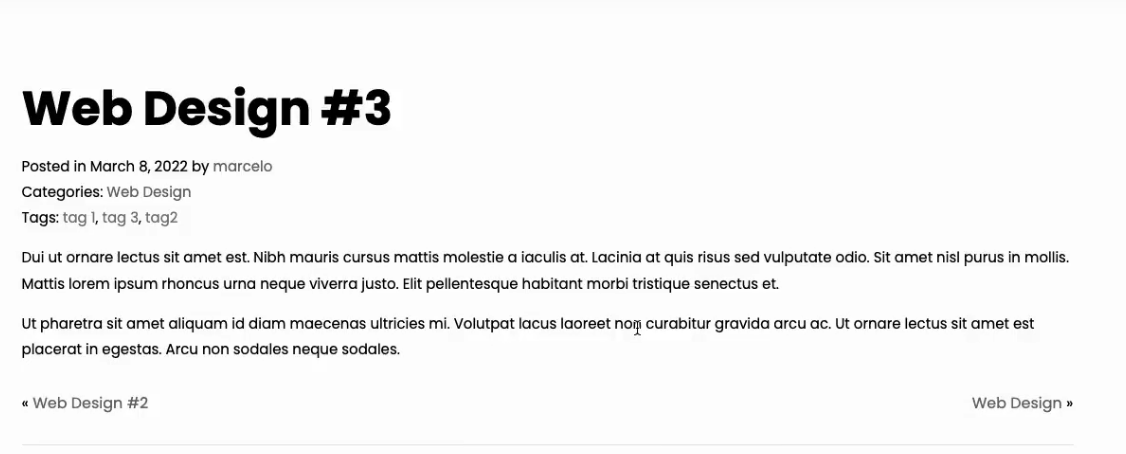
3. Numerical Pagination for Search Pages
<div class="pagination">
<?php
the_posts_pagination(array(
'mid_size' => 2,
'prev_text' => '« Prev',
'next_text' => 'Next »',
'screen_reader_text' => ' ',
));
?>
</div>
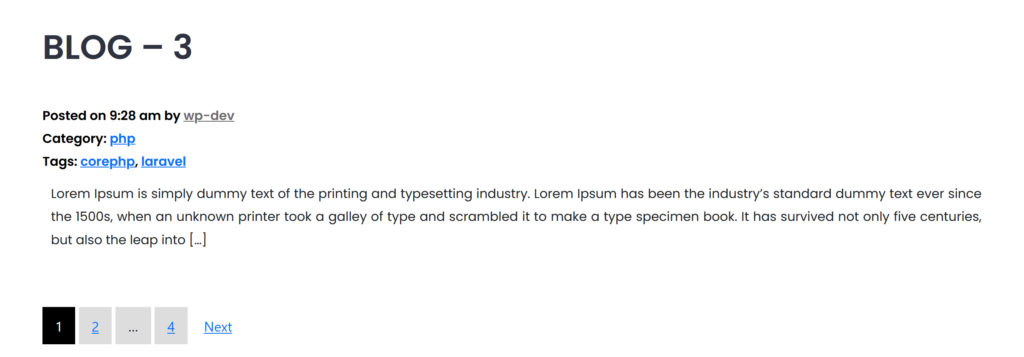
/* Add Pagination Styling in style.css or custom css */
.navigation, .post-navigation, .pagination {
display: flex;
justify-content: center;
align-items: center;
margin: 20px 0;
gap: 10px;
font-size: 16px;
}
.navigation a, .post-navigation a, .pagination a {
display: inline-block;
padding: 8px 12px;
text-decoration: none;
background-color: #0073aa;
color: #fff;
border-radius: 4px;
transition: background-color 0.3s ease;
}
.navigation a:hover, .post-navigation a:hover, .pagination a:hover {
background-color: #005f8d;
}
.pagination .current {
background-color: #ddd;
color: #333;
font-weight: bold;
padding: 8px 12px;
border-radius: 4px;
pointer-events: none;
}
.pagination .disabled {
background-color: #f4f4f4;
color: #999;
pointer-events: none;
padding: 8px 12px;
border-radius: 4px;
}